How to achieve website effects using jQuery.addClass()
The jQuery.addClass() method is a precise and user-friendly way to add classes to elements so that you can control the appearance and performance of your website. We’ll show you the structure of .addClass() and how to operate it.
What is the jQuery.addClass() method?
By using .addClass(), you can dynamically change the appearance of your website. By adding your desired classes to the appropriate HTML elements you can customise styles, design animations, or respond to user interactions. And to remove existing classes you can use jQuery.removeClass(). After adding the class, you can apply its individual CSS style rules to the selected elements. By using the .addClass() method you can achieve the visual performance you’d like for your website. Additionally, you can work with jQuery in WordPress to create custom layouts and effects in your CMS.
Rent your personal webspace from IONOS and benefit from at least 50 GB for your web project. You’ll also receive a free SSL wildcard certificate plus domain. Choose the right package with customised features.
What are the syntax and parameters of the jQuery.addClass() method?
The jQuery.addClass() function accepts two parameters. The general syntax is:
$(selector).addClass(classname,function(index,currentclass))
jQuery- classname: name of the class or classes to be added separated by a comma.
- function(index,currentclass): an optional callback function that will be called for each selected element
- index: the index of the current element in the selected element list
- currentclass: the current class of the element
The Callback function facilitates further customisation or logic at the level of each element while you add classes. This way you can check the current class value and add more classes depending on it.
If you are just getting started with jQuery, we recommend the jQuery tutorial in our guide, where you can learn all the basics of the jQuery library.
The IONOS API allows you to configure your IONOS hosting products quickly and easily. With the IONOS APIs access key, you can manage your domains, DNS settings and SSL certificates. For more information, see the document on the IONOS Developer API info page.
An example of using the jQuery.addClass() method
We’ll show you the function of .addClass() by adding the class ‘.green’ to the following p element. This will change both the colour and the font size of the paragraph. The change is triggered by a click event. Other methods that can be used with .addClass() are jQuery.find() or [jQuery.ajax()](https://www.ionos.co.uk/digitalguide/websites/web-development/jquery-ajax/=.
<!DOCTYPE html>
<html>
<head>
<style>
.blue {
color: blue;
font-weight: bold;
}
.green {
font-size: 24px;
color: green;
}
</style>
<!-- Import jQuery CDN library -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script>
$(document).ready(function () {
$("button").click(function () {
$("p").addClass("green");
});
});
</script>
</head>
<body style="text-align: center;">
<h1>
This is a jQuery.addClass() example.
</h1>
<p class="blue">
jQuery add class to element demo
</p>
<button>Click!</button>
</body>
</html>
htmlYou can see in the browser window that the colour and size of the p element changed after clicking the button. Before:
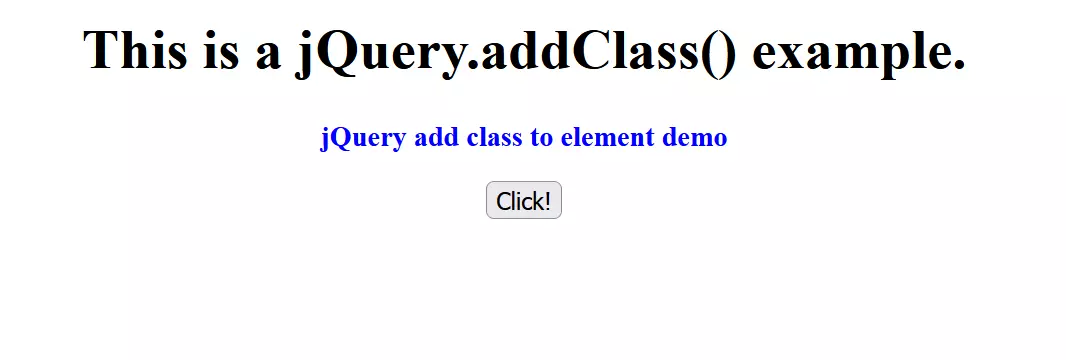
After clicking the button:
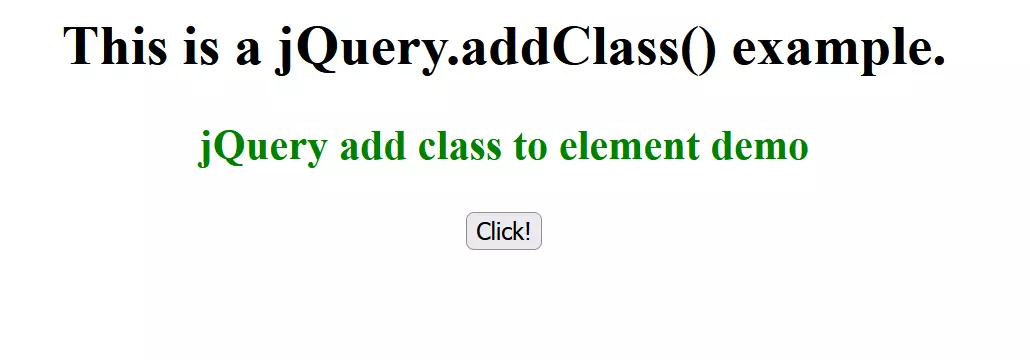
Get your project online with Deploy Now from IONOS. The quick setup and automatic framework detection means you can realise your internet presence in just a few simple steps. You can even sign up for additional resources on top of your Deploy Now membership. Additional subscriptions can be cancelled on a monthly basis. IONOS guarantees 100% customer focus with Deploy Now.